React Custom Hooks: What They Are and How to Use Them
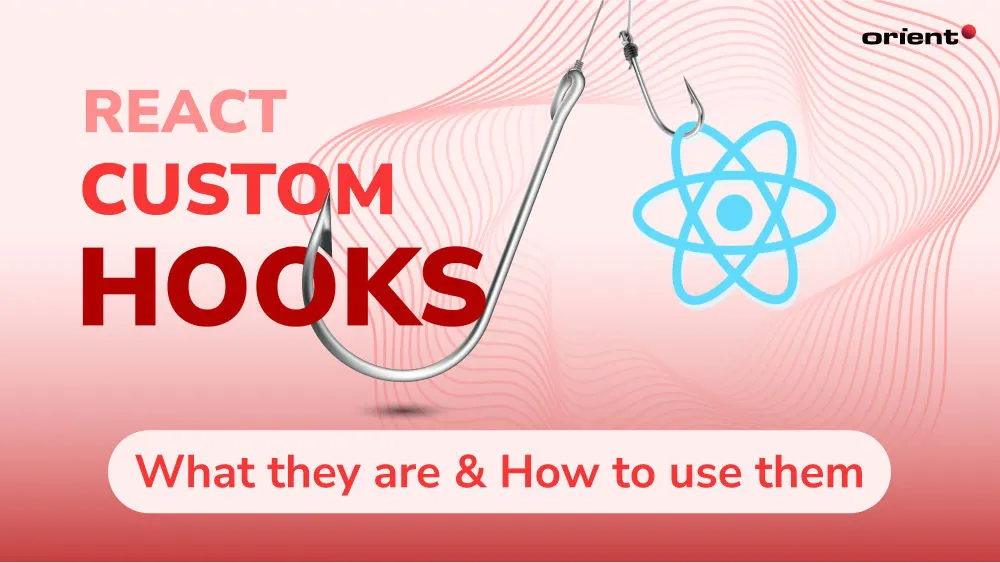
Content Map
More chaptersReact custom hooks help improve the quality, readability, and maintainability of React.js code. They are useful in the absence of suitable third-party libraries and React hooks. Understanding how React custom hooks are used can, in part, help you choose the right React.js development team for your project.
In this article, we discuss what React custom hooks are, their advantages, and how they are used in React development.
Key Takeaways
- React custom hooks are reusable functions for React applications
- React custom hooks help improve code quality by improving readability and maintainability.
- React.js developers that use React custom hooks may deliver higher-quality software in less time than those that do not.
What Is a React Hook?
Before we discuss React custom hooks, it is worth explaining how they came about. React Hooks are a feature added to React version 16.8. Hooks allow functional components to access state, lifecycle methods, and other React features.
- Functional components are JavaScript functions that return JavaScript XML (JSX) elements rendered to a user interface (UI).
- A state object stores property values that belong to a React component.
Before React 16.8, only class components could access the state, becoming ‘stateful.’ Stateful components can change over time, typically in response to the user’s actions and other external factors. Meanwhile, stateless components have no state or lifecycle methods, as they primarily present static data and UI elements.
React Hooks simplifies state management, allowing developers to render virtually all UI elements with functional components, making class components largely obsolete. The most common React hook commands are:
- useState: Manage component state
- useEffect: Handle side effects in functional components
- useContext: Access the value of a React context
What Is a React Custom Hook?
React custom hooks are reusable functions that add special or unique functionalities to React applications. Typically, developers use third-party libraries or React Hooks to add unique functionalities. However, when these solutions do not suffice, developers resort to using custom hooks. There are other reasons, outside of pure necessity, to use custom hooks.
By encapsulating code snippets into custom hooks, React.js developers can reuse the same logic across components and React UI frameworks, resulting in cleaner, more concise, and easier-to-read code. Consolidating can be done at any stage of development. For instance, if developers find repetitive logic across components, they can extract that logic to a custom hook, converting several lines of repetitive code into a single phrase.
Like React Hooks, custom hooks begin with the phrase “use,” followed by the developer’s chosen naming convention. For instance, a custom React hook could be “useOnline,” with the intent to track whether a user is online. Custom hooks allow for more functionalities beyond the previously mentioned React Hook commands (useState, useEffect, useContext).
What Are the Advantages of Custom Hooks?
React custom hooks help promote code reusability, improve code readability, and streamline code maintenance. These benefits are great for React.js developers, sure. But these benefits also extend to clients – as in, you. By outsourcing your React.js development to a team using advanced techniques, you’ll likely receive a higher-quality product in less time.
Promote Code Reusability
When React.js developers create custom hooks, they can reuse the logic in those hooks across React function components. This approach helps reduce manual coding, as developers can copy and paste custom hooks into their code faster than manually writing the logic for a function each time. Aside from making code cleaner and more concise, the code will also render faster, reducing unnecessary waiting.
Aside from promoting code reusability, custom hooks help enforce software development standards for a development team. This helps make the code more consistent and easier to predict the code’s behavior. This is one of many reasons why React is so popular for front-end UI development.
Improve Code Readability
React custom hooks improve code readability by eliminating unnecessary boilerplate code. Instead of comprehending several lines of code, developers need only understand the logic behind a single phrase. For instance, if a developer sees the previously mentioned “useOnline” hook, they know the purpose of that hook is to track whether a user is online.
Let’s say you hire a development team to create a banking app. For security reasons, you request a feature that automatically logs out an inactive user. After creating the logic for this feature, the team encapsulates that logic into a custom hook so they can use it across the entire app. By reducing unnecessary code, the code loads faster and is easier to read.
Streamline Code Maintenance
React apps with other hooks can help streamline code maintenance. Let’s say you need to update a feature. Sure, a developer could manually rewrite each instance of that logic, but that would be time-consuming. Instead, the developer can update the logic within a custom hook and update each instance of that hook – replacing the old with the new.
Aside from streamlining maintenance, custom hooks also reduce the risk of introducing new bugs.
When to Use React Custom Hooks
There are many reasons to use custom React hooks. The most common use case is to create a hook that serves a very specific purpose, to be exact, a purpose that a developer cannot achieve with a third-party library or standard React Hook. The other use case is to reuse a custom hook across React components in the same app.
Here are two hypothetical case studies that demonstrate when to use React custom hooks.
Share Logic Between Multiple Components
You request a feature that shows a user their network connection status. The developer creates a state, tracking when the network is online, and an Effect, denoting the state as online or offline. The developer successfully implements the network connection feature in the app.
Then, you request a new feature, the ability for a user to save their settings. However, the user can only do this while online. To save time, the developer encapsulates the component logic of the previous feature into a custom hook. They use this custom hook to power a new component, a button that says ‘Save’ when the user is online and ‘Reconnect’ when the user is offline. This way, the button changes its appearance and separate function in response to the user’s network status.
Hide Complex Logic
You request a new feature that lets a user copy text to a clipboard. When the developer types out the stateful logic for this feature, it occupies about 27 lines of code.
To improve code readability, the developer stores the logic of this feature into the custom hook phrase “useCopyToClipboard.” The developer uses this custom hook four times during development. So, instead of writing 108 lines of code, the developer writes only four lines to achieve the same outcome.
Read more: Programming Logic for Beginners
Best Practices When Using Custom Hooks
Are you outsourcing your React.js development? If so, it’s worth being familiar with the best practices that React developers follow. This way, you can accurately assess the quality and efficiency of a potential team. Here are the best practices that most developers follow for custom hooks.
Make Each Custom Hook Single-purpose
Each custom hook should, ideally, have only one purpose. This makes it easier to duplicate across compartments. If a custom hook is multi-purpose, it may be harder to reuse in a functional component. If necessary, split up a multi-purpose custom hook into single one-purpose hooks.
Use Dependencies
Some custom hooks rely on external libraries or contexts to function. In this case, developers should add dependency injections to these custom hooks so they can accept essential dependencies.
Write Documentation and Comments for Hooks
When creating custom hooks, developers should write documentation and comments explaining:
- The purpose of the custom hook
- The technical specifications (parameters, return values)
- How to use the custom hook
By providing this information, developers can quickly ascertain what a custom hook does and how it works within the app’s context. This benefit also extends to developers who may be unfamiliar with the code.
Custom Hooks for Better Code Quality
Custom hooks are a powerful way to extend React app functionality. They also help improve code readability and streamline maintenance. For these reasons, choose a React.js development team that understands how to use this helpful feature.
For over a decade, we here at Orient Software have provided React.js development services for clients. Our React apps are user-friendly, extremely lightweight, and easy to update, maintain, and personalize. Plus, we guide you through each step of development so that you know what to expect.
You can learn more about our React.js development services by contacting us today.